Base64
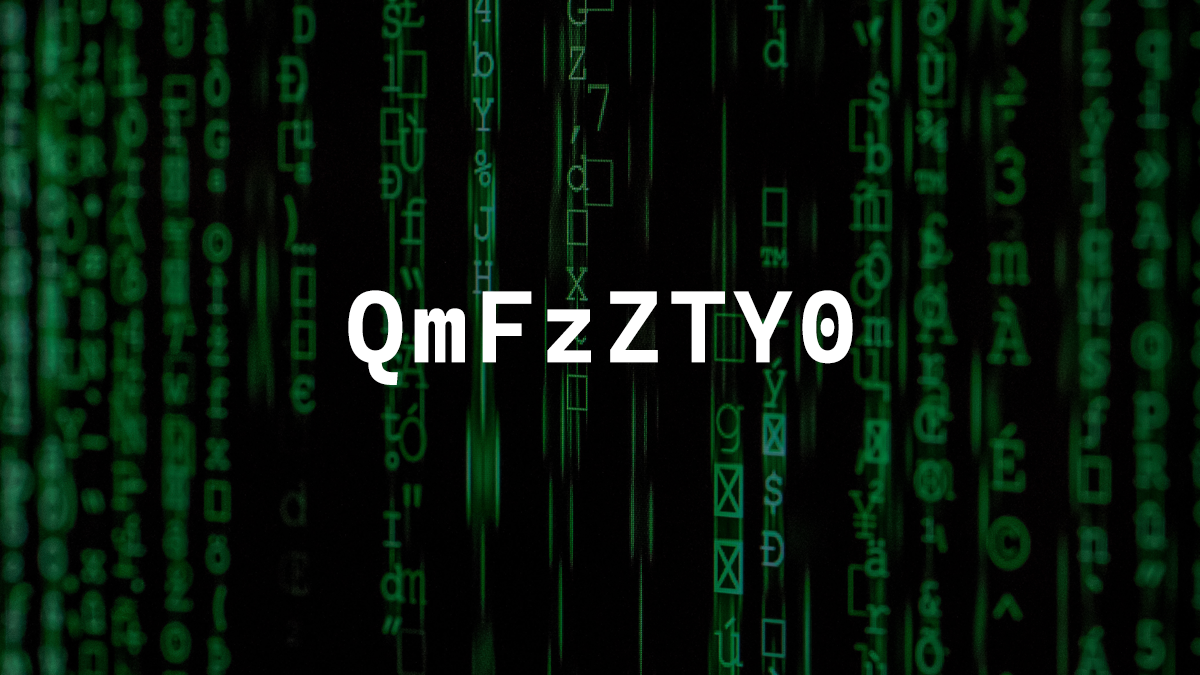
Base64 is a form of binary-to-text encoding that is very widely used, you can encode almost anything into Base64. Learning how to leverage Base64 has been a major eye-opener for me.
I’ve seen Base64 used in countless places such as SSL certificates. Ransomware loves to use it, as a lot of basic Antivirus don’t check to see what’s happening in a Base64 string and ignores it. You can encode an entire PowerShell command with Base64 and just run PowerShell with the -EncodedCommand
option to run something that a basic Antivirus might not notice.
Base64 is also widely used in phishing emails, be it the URL or even the username or the entire phishing page being an HTML attachment in an email and everything is in Base64.
Even though there are malicious things that can be done when using Base64 to obfuscate or even double obfuscate things, there are genuine day-to-day use cases.
Let’s say you need to install a program but you need to use a transform file. The vendor might host the installer publicly but what do you do about the transform file? You could host it somewhere but maybe the transform file has specific information that shouldn’t be public like the SKEY and IKEY for a Duo install.
A solution could be to take the transform file and encode it with Base64 and put that in an install script. Now everything is self-contained. Yes if someone gets ahold of the install script they also have the transform file info. However, I feel like the risk for that is less than publicly publishing the transform file be it an obscure URL or not.
Another use could be if you wanted to have a self-contained error page but you want the error page to have your logo and be completely independent of any external resource, you could use Base64 to embed the logo into the page.
An example of this is Password Pusher they pulled a commit of mine that tweaked the images on the error pages to be fully self-contained. Here’s an example of one of the error pages https://pwpush.com/error
If you look at the source code you will see that the logo is stored in Base64.
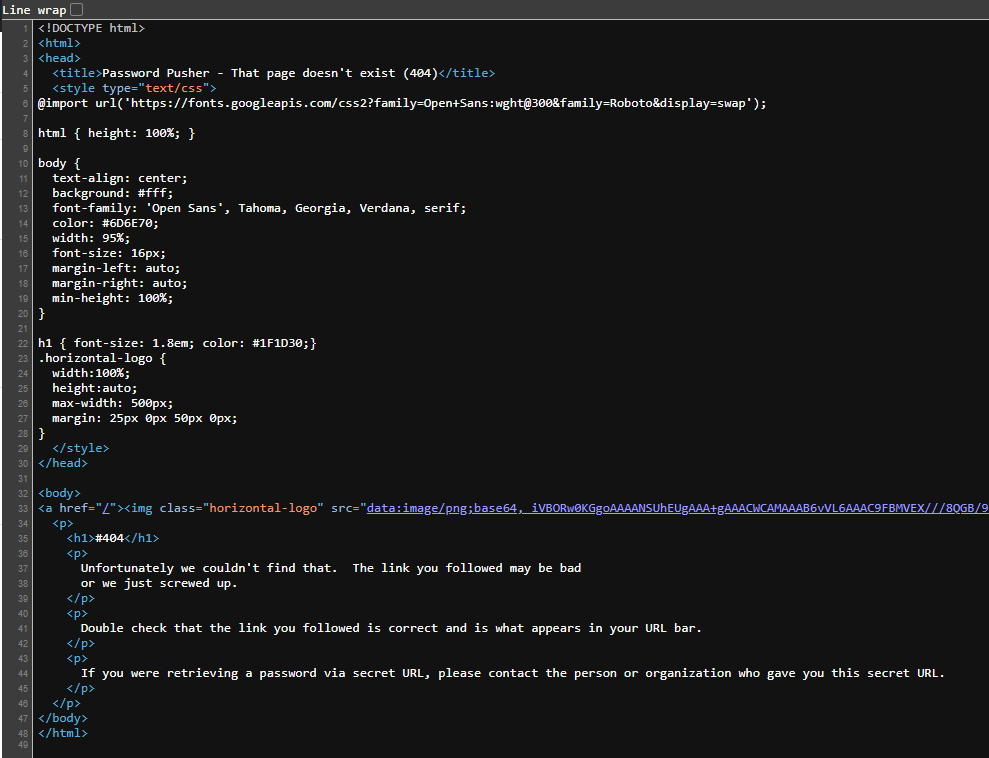
As you can see the logo is fully encoded with Base64 making it self-contained to the page itself.
PowerShell
There are countless times when I’ve put Base64 directly into my PowerShell scripts. Here are some of the PowerShell scripts I created that I tend to use a lot as functions in other scripts.
Encoding a File
The PowerShell code below will encode any file into Base64 and copy the output to the clipboard.
$file = "C:\temp\file.txt"
[convert]::ToBase64String((Get-Content -path $file -Encoding byte)) | Set-Clipboard
Code language: PowerShell (powershell)
I have a text file named file.txt in C:\temp\ once I encode that file with Base64 I now have a value of SSBhbSBmaWxl
for the file. That is the Base64 string for the whole file and the contents of that file.
Decoding a File
Now I’ll output the Base64 encoded file into a new file named file_B64.txt
Below is the PowerShell code to take a Base64 encoded file string and output it to a new file.
$file_B64 = "SSBhbSBmaWxl"
$Dest = "C:\temp\file_B64.txt"
$out = [Convert]::FromBase64String($file_B64)
[IO.File]::WriteAllBytes($Dest, $out)
Code language: PowerShell (powershell)
If you run that you will get the same file and its contents that I used for this example.
Encoding Text
Below is the PowerShell code to encode text into Base64 and copy the output to the clipboard.
$text = "Some Random Text"
[Convert]::ToBase64String([System.Text.Encoding]::UTF8.GetBytes($text)) | Set-Clipboard
Code language: PowerShell (powershell)
Here is the output for the PowerShell code above U29tZSBSYW5kb20gVGV4dA==
Decoding Text
Below is the PowerShell code to decode Base64 encoded text and copy the output to the clipboard.
$text_B64 = "U29tZSBSYW5kb20gVGV4dA=="
[System.Text.Encoding]::UTF8.GetString([Convert]::FromBase64String(($text_b64))) | Set-Clipboard
Code language: PowerShell (powershell)
Here is the output for the PowerShell code above Some Random Text
Final Thoughts
You can do a lot with Base64, I’ve only just scratched the surface. The more I use it the more uses I find for it. It’s shocking how many items are actually Base64. Whenever I see a string of something that looks to be random numbers and letters I try and decode it to see what it actually is.
If you want to read more about Base64 here is RFC 4648 about Base64.
Leave a comment